Turning a Raspberry Pi into an expensive light timer
A simple beginner project to use the Raspberry Pi and Piface board to automatically turn things on and off.
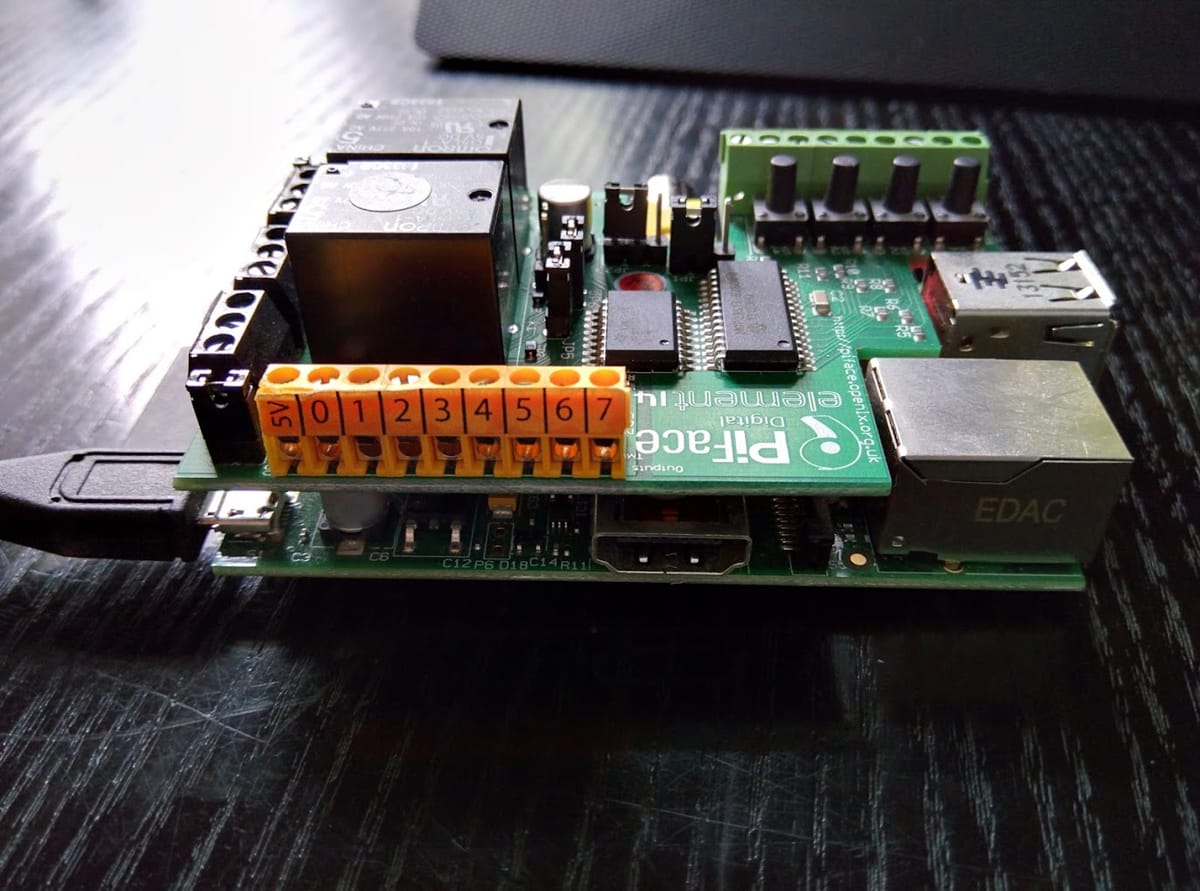
The Kit
About a year ago, I got my first Raspberry Pi. It was a model B.
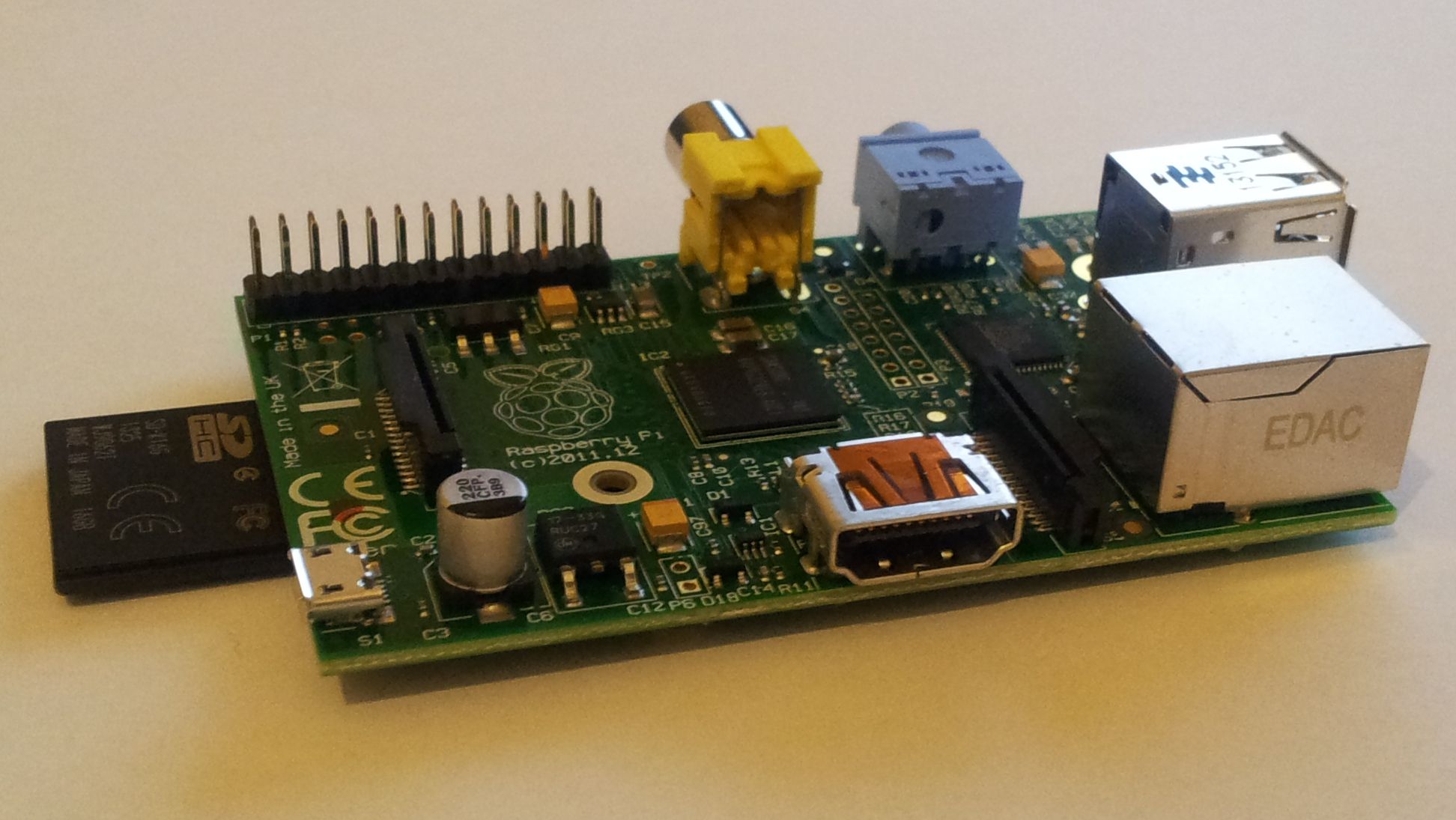
I also bought the PiFace board with it as I thought it would be quicker and easier to control things and I could use Python, which is my favourite language.
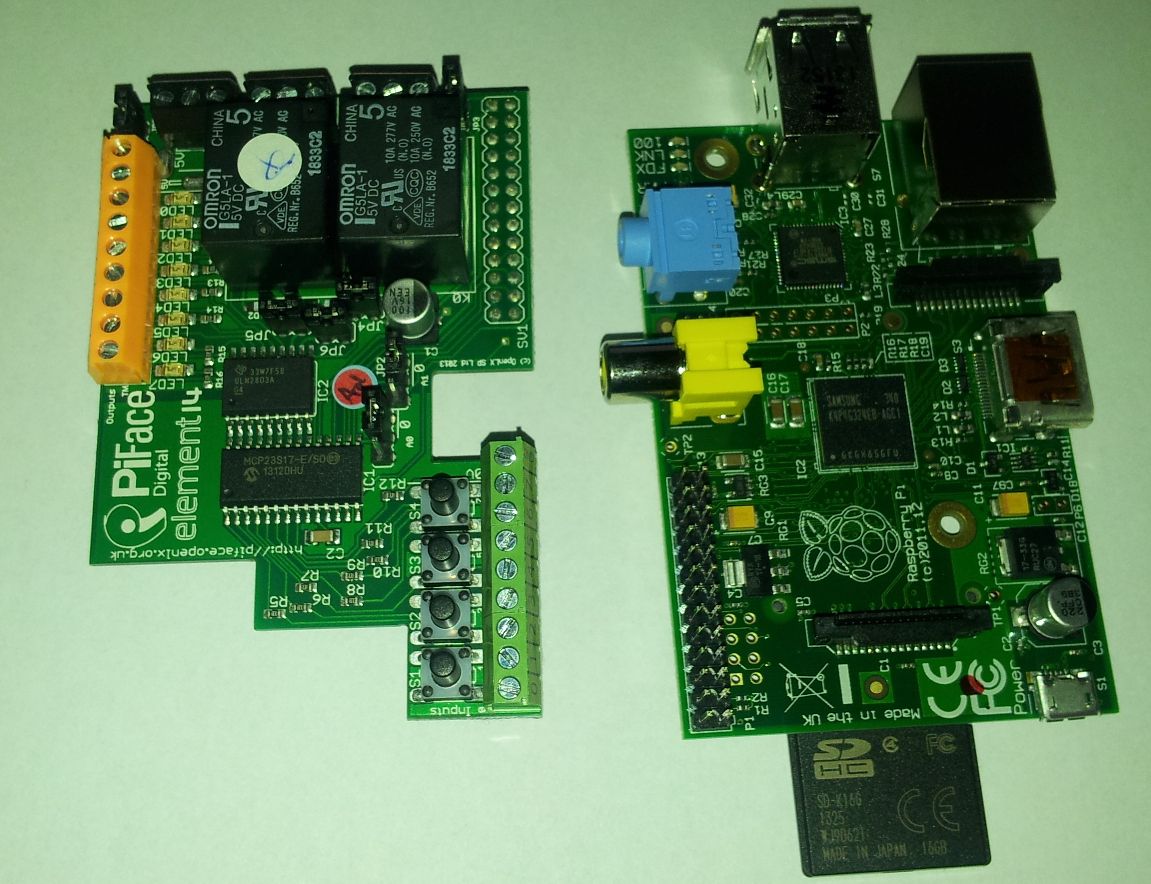
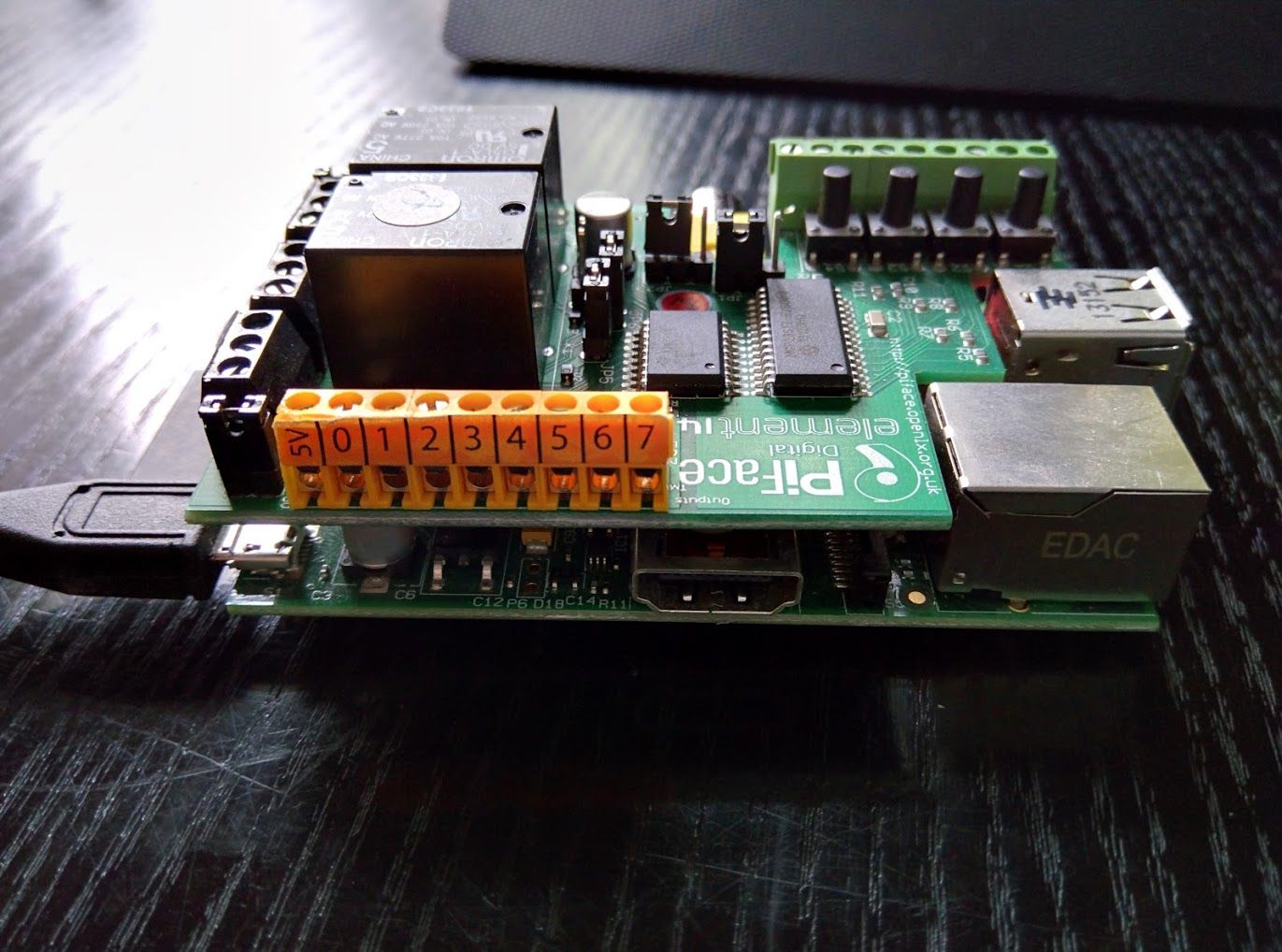
I found out later that you can use Python to control the Raspberry Pi without the PiFace board, but I already had it by then and it was the easiest way for me to start messing with electronics.
I am not as strong at the electronics side of things, so this seemed like a good way to hit the ground running and start turning things on and off.
The Project
If you didn't notice from the cover page, I am a massive fan of snakes and in particular, the Green Tree Python. This project is actually for the one that is pictured on the cover page.
The issue was that its cage was quite dark during the day, so I needed a light in there.
The lighting had to meet the following requirements:
- The cage wasn't very high, so I needed something compact
- I needed something cool that wasn't going to burn the snake
- It needed to either be robust enough for the snake to climb onto or not allow the snake to climb onto it at all.
- I needed it to turn on automatically in the morning and off automatically at night
With these in mind, I settled for an LED strip. I grabbed a 5m spindle of waterproof white SMD LEDs from ebay and I was ready to go.
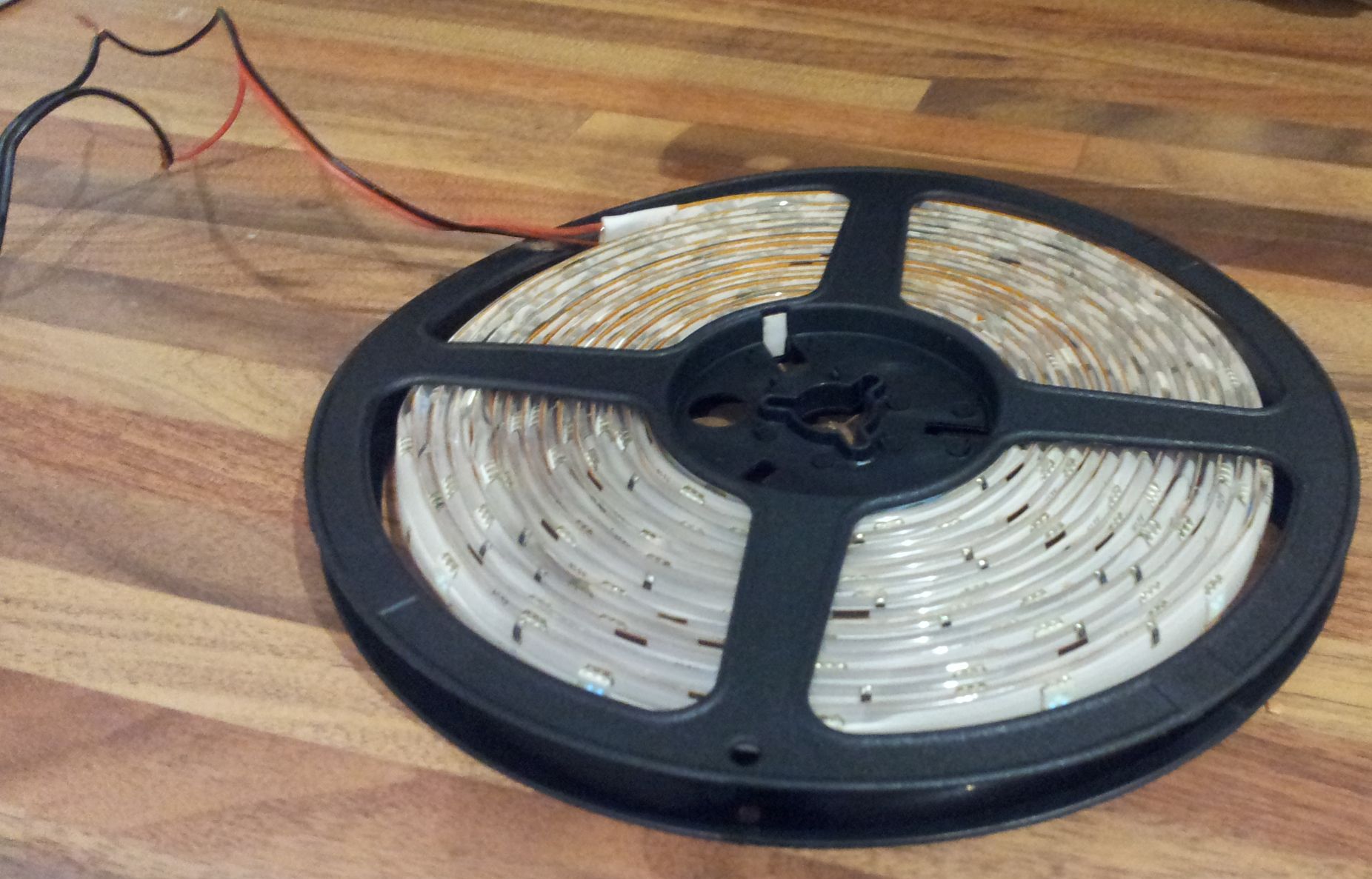
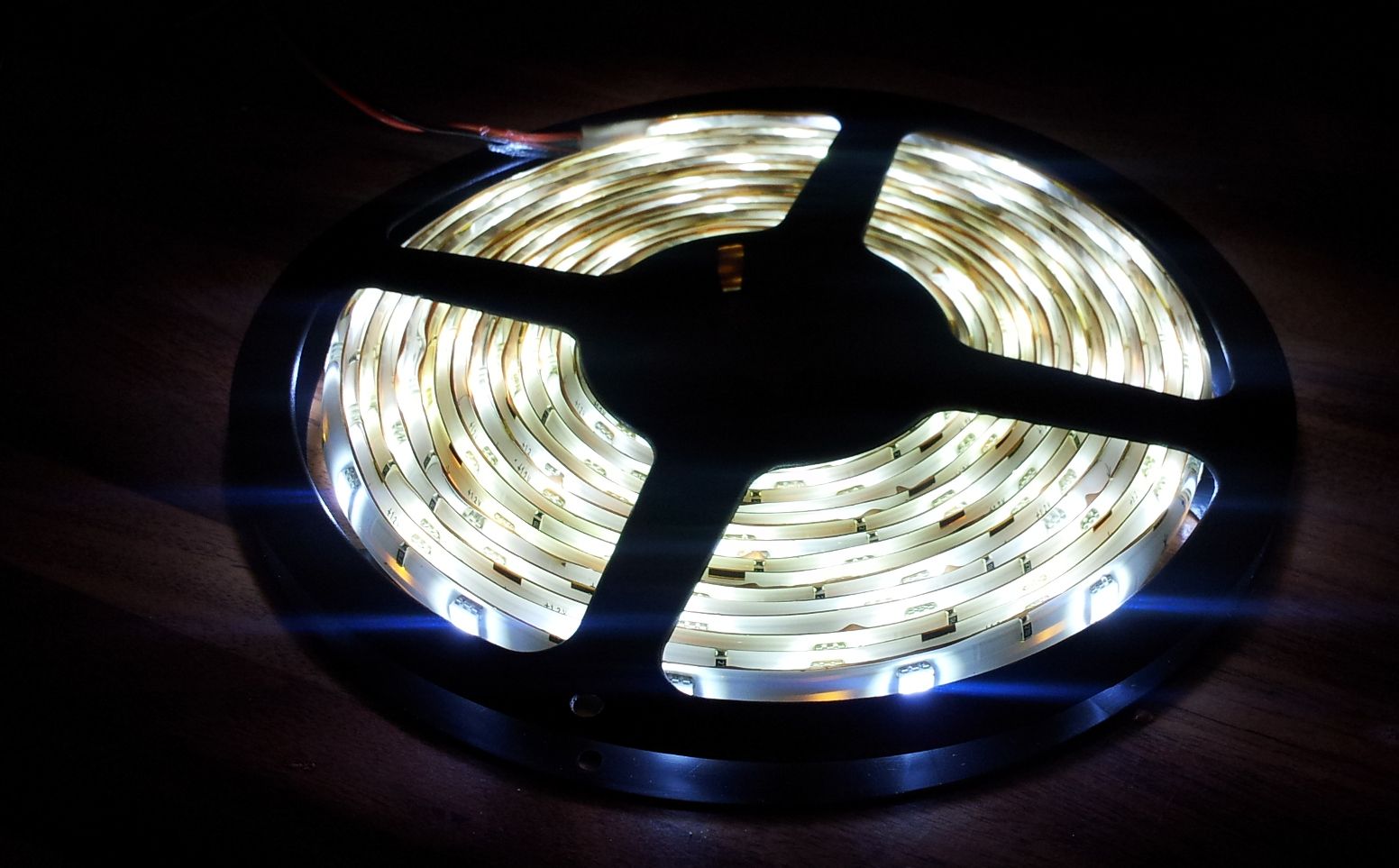
I only needed about 20cm of the strip and I installed it in the cage and ran it directly from a 10V DC power supply. The effect in the cage was quite satisfying.
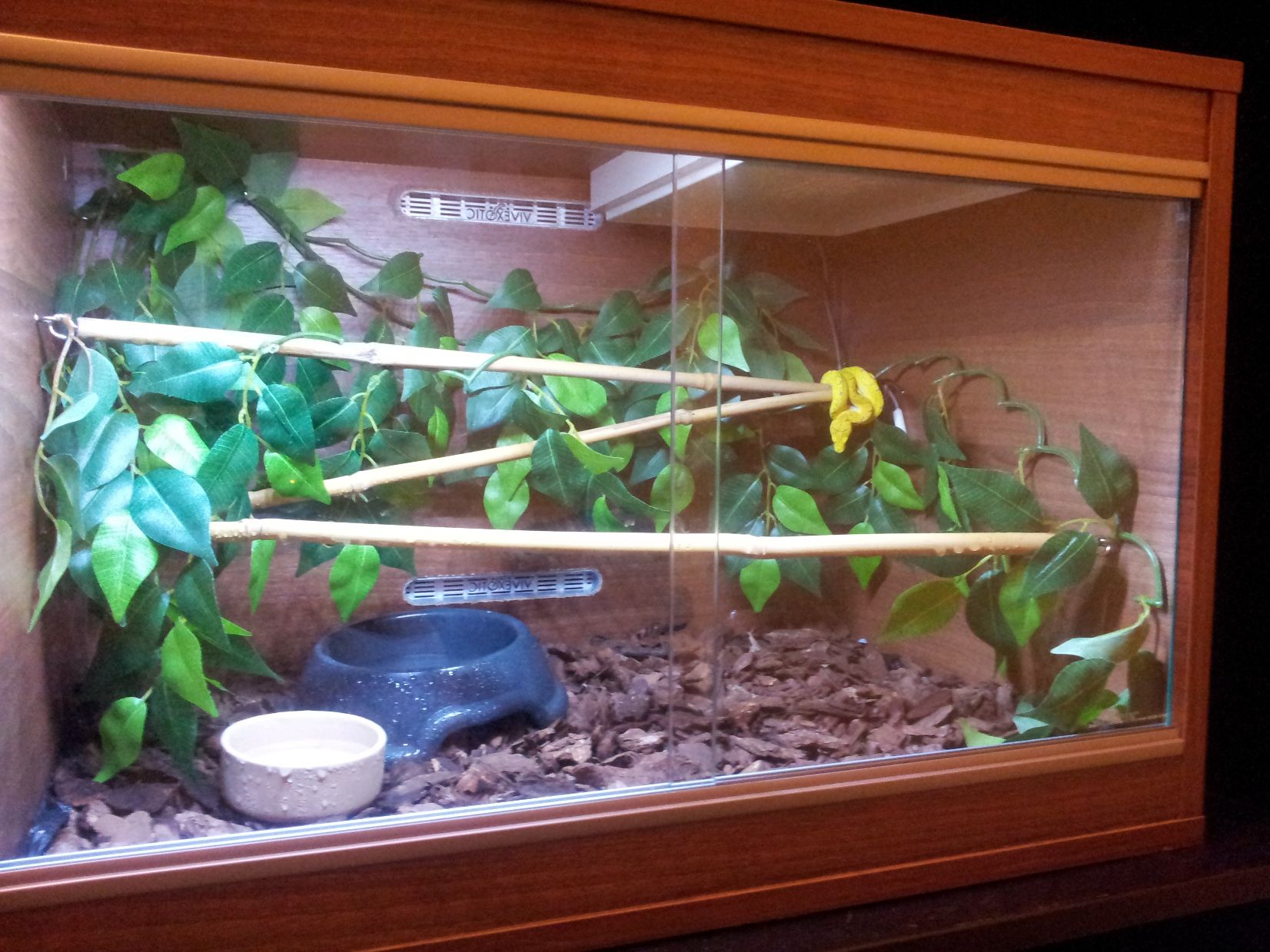
The programming part
So with the lights working and in place I needed a way to automatically switch them on and off.
I could have used a simple plug timer that would have cost less that £5 and be done with it...

...but I already had the Raspberry Pi and the PiFace board and I was determined to put them to good use.
The wiring was fairly straight forward. Since I was using DC current, I could use the normal output pins and didn't have to worry with the noisy relays. The positive from the power supply went to the LED strip and then to output pin 7 on the PiFace board and the negative came from the output ground pin and back to the power supply.
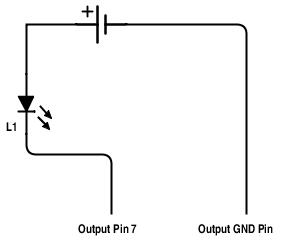
Note: Since I was using a current higher than 5V, it was necessary to remove jumper number 4 from the PiFace board to disconnect the built in snubber diodes. When using 5V the snubber diodes protect the driving transistors from voltage spikes, but if a higher voltage is used, apparently the diodes will conduct between the outputs and the 5V circuit.
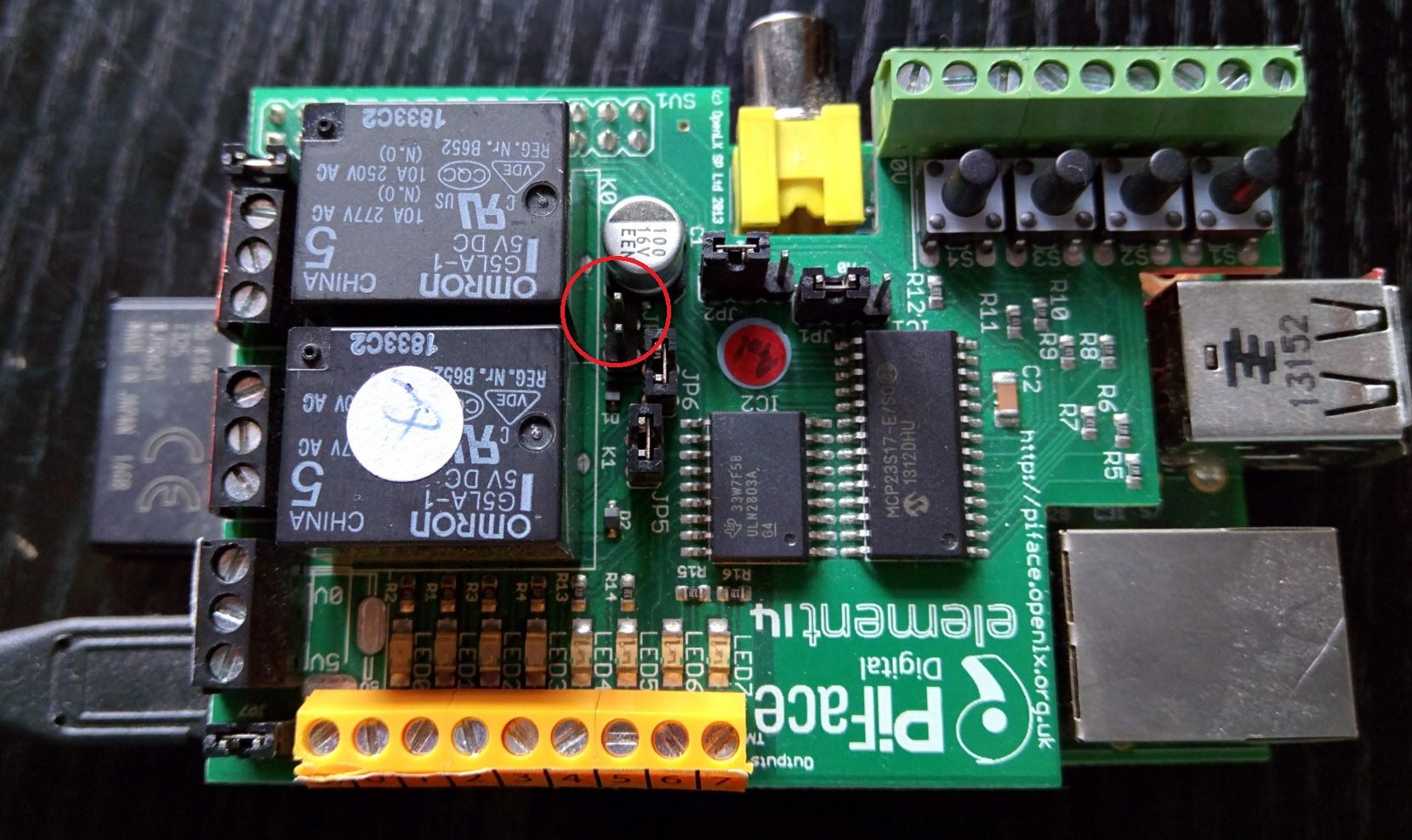
Next I installed the pifacedigitalio library on the Pi after a bit of messing around. Only the Python 3 version worked for me.
Once I had played with the library, cycling the outputs etc., I had to come up with a plan for how to automatically turn it off and on.
I first thought of creating a Daemon to check the light cycles in the Papua region of Indonesia, where this type of snake originally comes from. I would take these light cycles and offset them by 6 months to try and match them to our seasonal light cycles. But after studying the light cycle over the year, I found that being so close to the equator, the light cycle only varied by about half an hour each way over the whole year.
So I scrapped that idea and went for the obvious, a cronjob that turned it on in the morning and a separate one that turned it off in the evening.
After looking into the pifacedigitalio I found that it has almost everything that I wanted already, all I needed to do was wrap it up in a python script so that it could be called from the command line in a single command, to make it easier to run as a cronjob.
This is the script, taken from the little project I made for it on GitHub
from pifacedigitalio import PiFaceDigital
from optparse import OptionParser
parser = OptionParser()
parser.add_option("-a","--action", dest="action", default="toggle", help="action to be taken: toggle, on, off. Default = toggle")
parser.add_option("-n","--number", dest="number", type="int", default=0, help="which number output to change: 0 to 7. Default = 0")
(opts, args) = parser.parse_args()
if opts.action not in ["toggle", "on", "off"]:
print('Action can only be "toggle", "on" or "off"')
exit(-1)
if opts.number > 7 or opts.number < 0:
print('Number can only be an interger between 0 and 7')
exit(-1)
pi = PiFaceDigital(init_board=False)
if opts.action == "on":
pi.output_pins[opts.number].turn_on()
elif opts.action == "off":
pi.output_pins[opts.number].turn_off()
else:
pi.output_pins[opts.number].toggle()
The script is simple enough, you execute the file using python and pass in the arguments for the action and the pin on which you wish to perform the action.
python3 pi_output_control.py -n 7
#toggle output number 7
python3 pi_output_control.py --number 6
#toggle output number 6
python3 pi_output_control.py -a on -n 5
#turn output number 5 On.
#if output number 5 is already on, nothing will happen.
Also, instantiating the object of class PiFaceDigital
with the key word argument init_board=False
meant that it preserved the current state of the board. This was important if I wanted to control more than one output at a time, using this method.
And that was it, you just run the command and it turned the light on and off.
There seemed to be a bit of a lag while python gathered all of its resources before executing, but it wasn't a problem for what I was using it for.
All that was left was to create the cronjobs
crontab -e
#access cron tab
#add the following to the cron tab to automatically call the sript at certain times
0 6 * * * python3 /path/to/script/pi_output_control.py -a on -n 7
#turn output 7 on at 6 am
0 18 * * * python3 /path/to/script/pi_output_control.py -a off -n 7
#turn output 7 off at 6 pm
Results
The cron, script, Raspberry Pi, Piface and LED strip combination worked really well for me, at first.
I ran it for 4 or 5 months before the LED strip gave out from being positioned next to the heat panel in the cage.
The LED strip had turned brown and brittle.
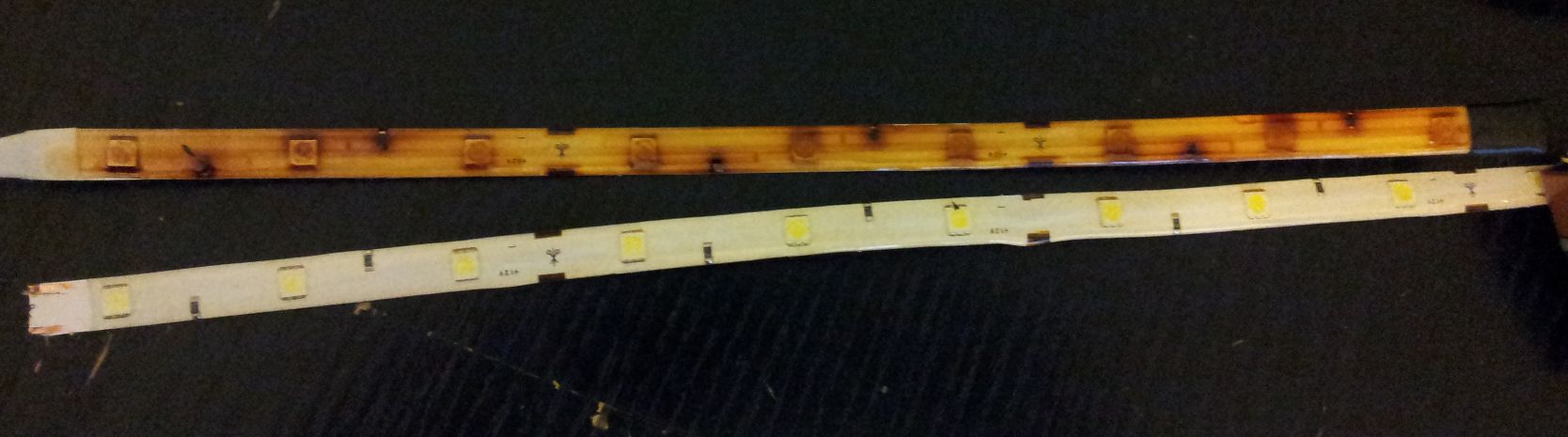
And it had become so dim, that I couldn't tell if it was on inside the cage or not.
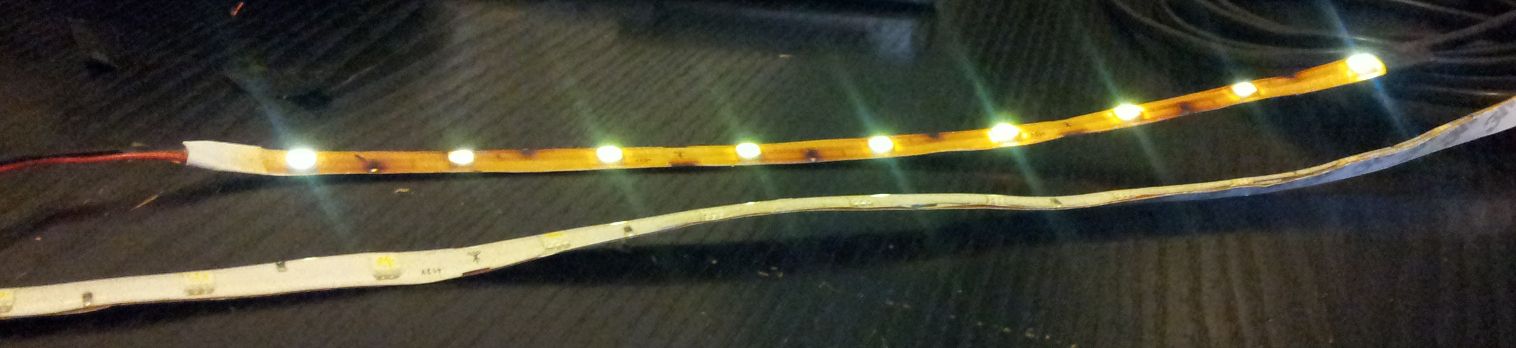
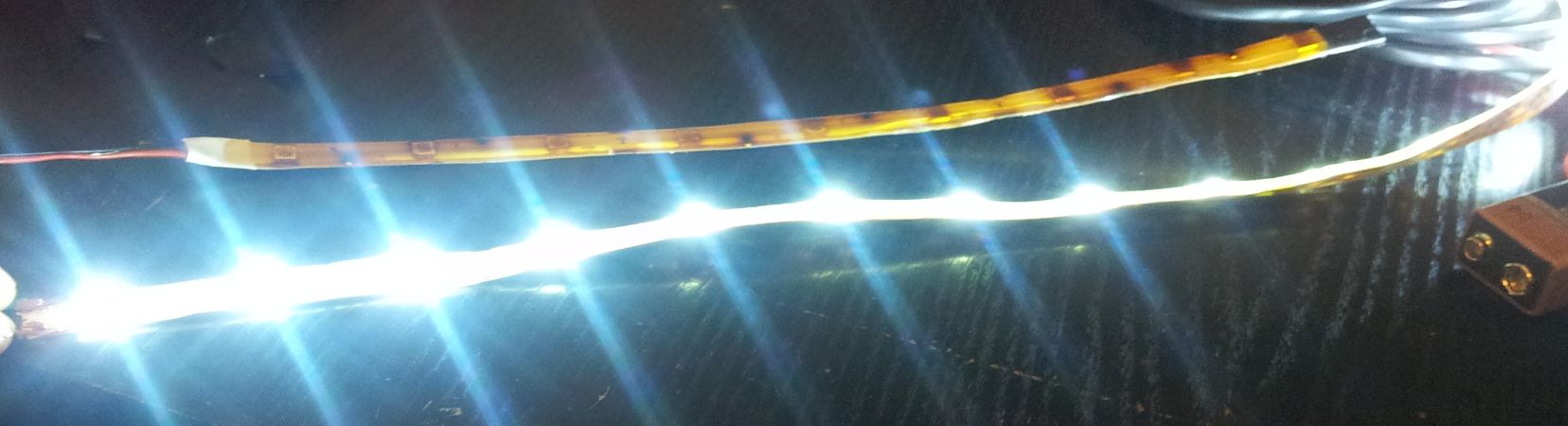
So in the end the cheap LEDs let me down, but the rest of the project worked fairly well.
If you need to turn something off or on and have too much time, a Raspberry Pi, PiFace board and couldn't be bothered to spend £5 on a timer plug, then this might be the solution for you.